Dictionaries in Python in 5 mins
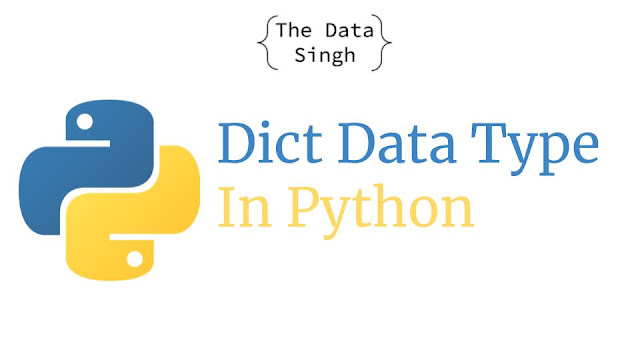
What is a Dictionary or Dict? A dictionary is a data type used to store key-value pairs as we have in our English dictionary. It is useful when we want to save the data related to an entity at the same place. It is like saving an excel row in python in a column_header:column_value format E.g If we want to store the details(name, class, marks, address) of a student we can create different variables for this, or we can create only one variable of type dict and save the values in it. Syntax: s1={ ‘Name’:’Tarun’, ‘Class’: 12, ‘Address’:’house number 11 xyz street ‘, ‘marks’:[90,89,67,84] } As in the above code, we can see that a dictionary can save multiple data types. Variable “Name” is of type string, “Class” is of type int, “Address” is of type string, “Marks” is of type list. A dict can also store a dictionary in it and is called Nested Dictionary. Syntax : <variable name>=dict({key:value,key:value}) <vari...